Game project 6-8:
The Crownest Editor
Overview
Crownest is a custom engine made for game project 5, 6, 7 and 8 at TGA. It is during project 6-7 that the editor was created and then during project 8 it was polished.
Inspector
Auto rendering for components
With the engines reflection/serialization system, the editor can render a custom inspector for every component in the engine. without having to write a custom inspector for every component. Allowing for quick iteration when wanting a variable you can change in the editor.
struct GridDescription
{
int MaxSubdivisionCount = 5;
float MinimumDetailDistance = 0.f;
float MaximumDetailDistance = 200.f * 100.f;
};
External_DOT_Definition(GridDescription, MaxSubdivisionCount, MinimumDetailDistance, MaximumDetailDistance)
struct DebugBuffer
{
bool EnableSkew = false;
bool EnableWind = true;
float SkewAmount = 0.f;
};
External_DOT_Definition(DebugBuffer, EnableSkew, EnableWind, SkewAmount)
class TerrainComponent : public ColliderComponent
{
private:
AssetRef<MeshAsset> myMesh;
AssetRef<MaterialAsset> myMaterial;
AssetRef<HeightmapAsset> myHeightmap;
GridDescription myGridDescription;
DebugBuffer myDebugBuffer;
float myDirectionalLightFactor = 1.f;
public:
Child_DOT_Definition(TerrainComponent, ColliderComponent,
myMesh,
myMaterial,
myHeightmap,
myGridDescription,
myDebugBuffer,
myDirectionalLightFactor
)
};
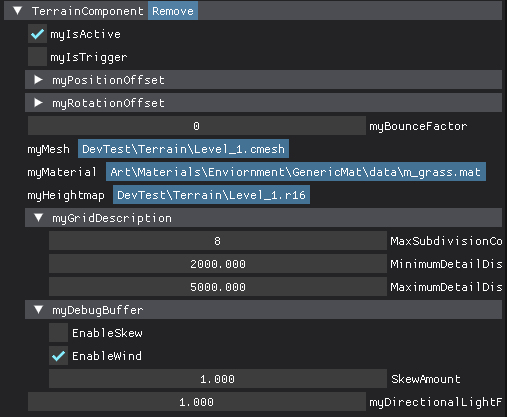
Used for inspecting all objects in the editor
The same inspector window is used for everything from gameobjects to materials, and it will render the correct inspector for the object you have selected.
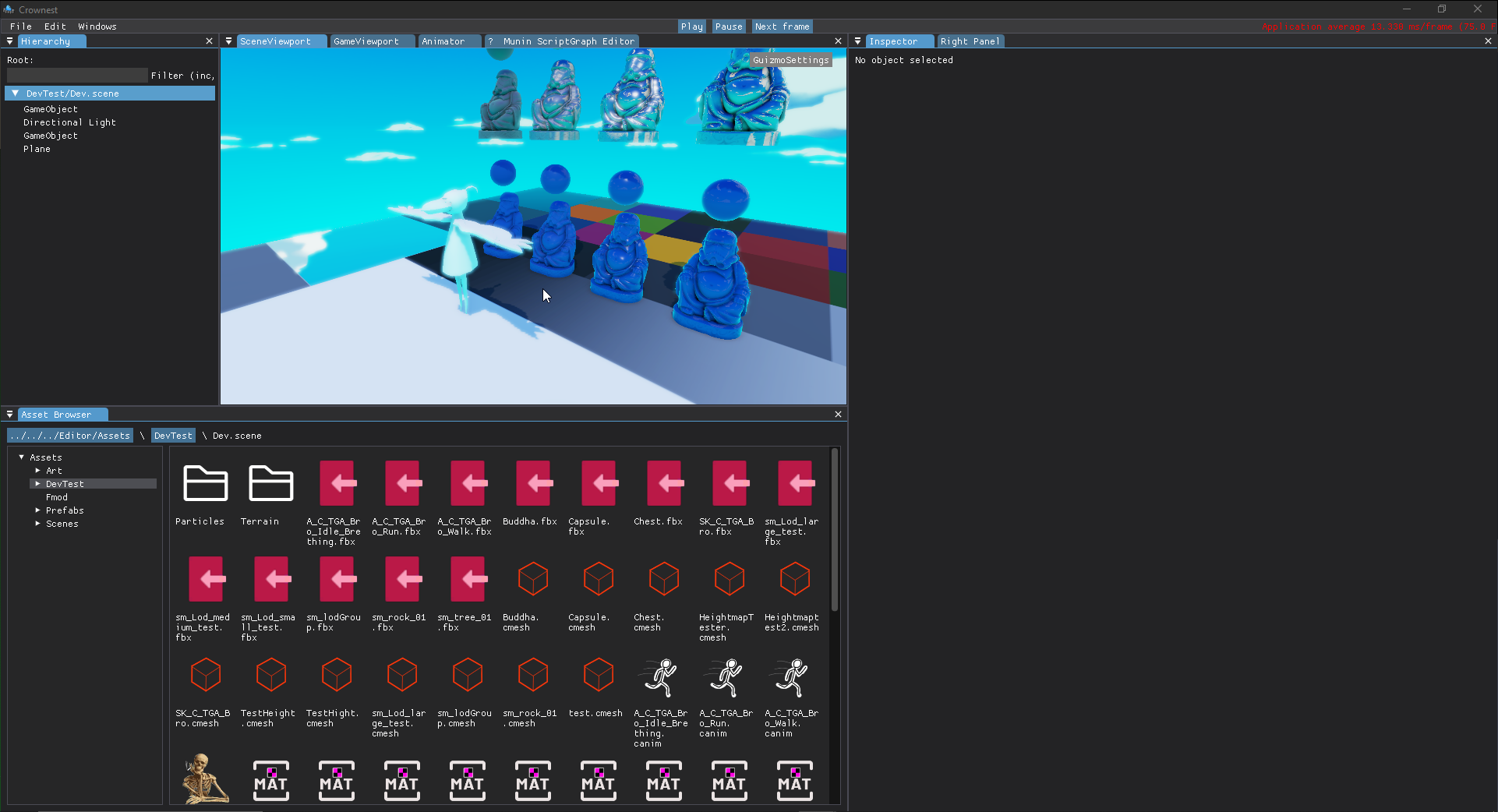
Hierarchy
Reparenting
Allows you to reparent objects in the scene, without the objects losing their position and rotation in the world
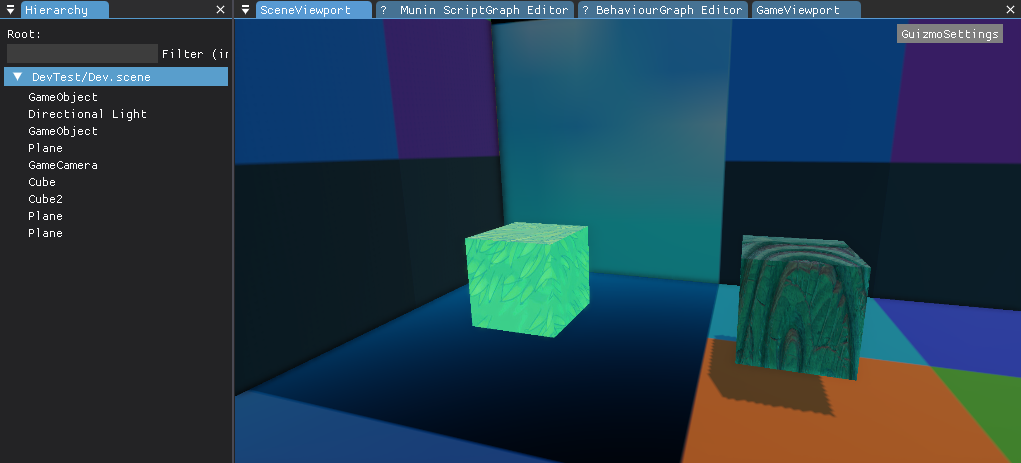
Search bar
Allows you to search for objects in the scene
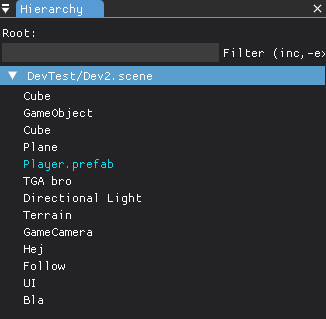
Playmode
Saves a temporary scene and allows for playmode, so the scene doesn't get changed when playing
Step frame
Allows you to step individual frames for debugging
Pause
Pauses the game
Scene and game view
Multi camera support
The Editor has Multiple viewports for the scene and game view, so you can see the game from different angles.
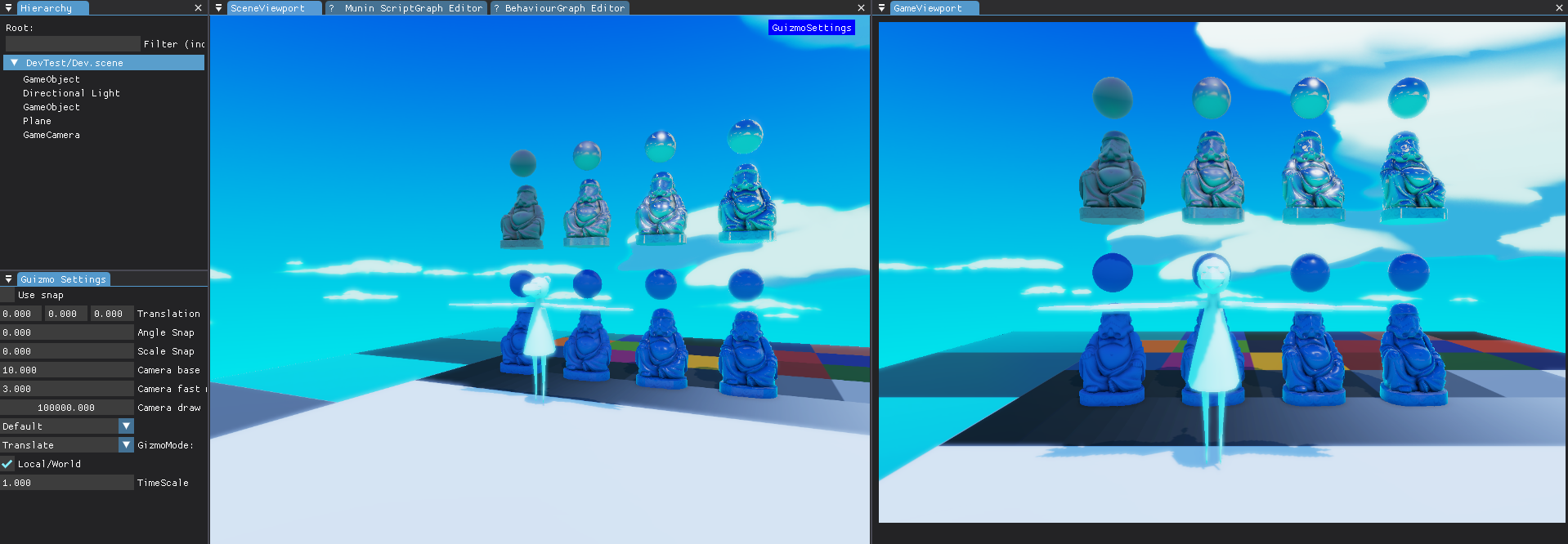
Prefabs
Live editing
When editing the prefab it updates in real time in the scene even before you save it.
Asset browser
Auto import capabilities
On the click of a button, the editor will import all assets in the asset folder, and then generate whatever type of asset that could be generate from those files, like generate a prefab for a model and then fill it with materials
Crash protection
If things in the scene crashes, then the editor will still be running and just tell you something went wrong, and let you continue as nothing happened. This feature was added, because it was way to easy for a small programmer mistake to make it impossible for level designers to continue working on the project. Until a quick fix was made. When the editor is started via visual studio it will crash so you can see the error message.
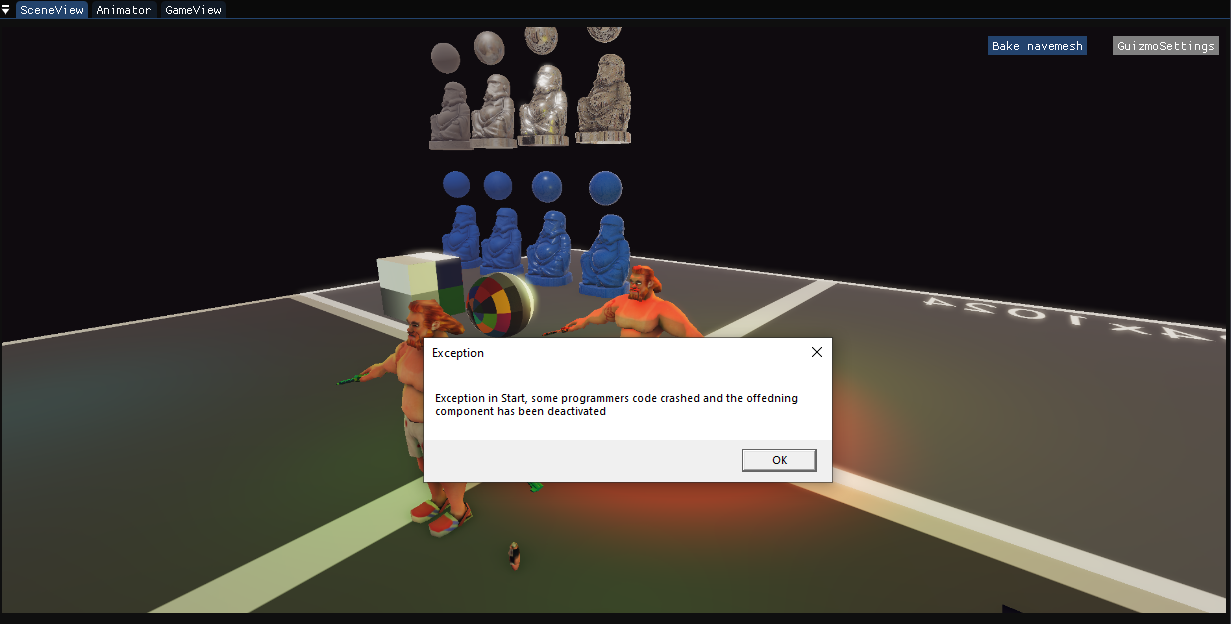
Gameobject system
Crownest uses a gameobject system, inspired by Unity.
Level system
The engine supports multiple scenes, and was used i project 8 to create a seamless world.
Serialization
The engine has a serialization system that can serialize and deserialize all components in the engine, and save and load levels. With a simple macro, you can easily select what variables your component should save.
class AnimatorComponent : public Component
{
private:
Nest::AssetRef<SkeletonResource> mySkeleton;
Nest::AssetRef<AnimatorAsset> myAnimatorAsset;
bool myShowSkeleton = false;
public:
Child_DOT_Definition(AnimatorComponent, Component, mySkeleton, myAnimatorAsset, myShowSkeleton)
};
Asset system
The asset system is a semi multi-threaded system, where you can request a asset and then only when you need it the main thread will wait for the asset to finish loading. So multiple assets can be loaded at the same time, without impacting the main thread.
Asset refs
The asset system has a reference system for being able to save references to specific assets so when a object with a asset ref is saved and then loaded it will try to load the asset it was refrencing without the programmer having to care about it.
class MeshComponent : public Component
{
private:
Nest::AssetRef<MeshResource> myMesh;
std::vector<Nest::AssetRef<MaterialAsset>> myMaterials;
public:
Child_DOT_Definition(MeshComponent, Component, myMesh, myMaterials)
};
Extra features
- FMOD hookin
- Custom Smart pointers (Built with game development patterns in mind)
- TGA Node scripting
- UI system (Canvas)
- Particle system